Animated Message
Create the illusion of animated messages on your
normal C++ output screen. This illusion will give the appearance that the code is being
"pulled" from an imaginary "hole" in the screen one letter at a time. These
messages can "pull"
out to the left or the right.
Message pulling to the left:
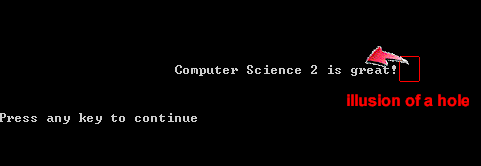
// message left
#include <iostream.h>
#include<stdlib.h>
#include "screen.h"
#include "apstring.cpp"
void banner_left(int length, int x, int y, apstring banner);
int main(void)
{
system("CLS");
apstring banner = "Computer Science 2 is great!";
// message for banner
banner_left(banner.length(), 50, 10, banner);
// function call
return 0;
}
// message left function
// function receives length of message, coordinates of hole, and message
//string function returns nothing
void banner_left(int length, int x, int y, apstring banner)
{
for (int i = 0; i < length; i++)
{
gotoxy(x-i,y);
// x and y are coordinates of the hole
for(int j = 0; j <= i;
j++)
{
cout<<banner[j];
}
delay(150);
// control the speed of the banner
cout<<flush;
}
cout<<endl<<endl<<endl<<endl;
return;
}
Message pulling to the right:
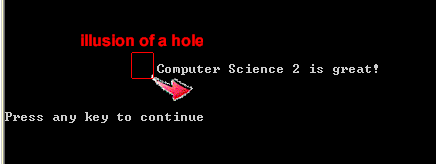
// message right
#include <iostream.h>
#include<stdlib.h>
#include "screen.h"
#include "apstring.cpp"
void banner_right(int length, int x, int y, apstring banner);
int main(void)
{
system("CLS");
apstring banner = "Computer Science 2 is great!";
// message
banner_right(banner.length(), 20, 10, banner);
// function call
return 0;
}
//message right function
// function receives length of message, coordinates of hole, and
message
//string function returns nothing
void banner_right(int length, int x, int y, apstring banner)
{
int k = 1;
for (int i = 0; i < length; i++)
{
gotoxy(x,y);
// x and y are coordinates of the hole
for(int j = length - k; j
< length; j++)
{
cout<<banner[j];
}
k++;
delay(150);
// control the speed of the message
cout<<flush;
}
cout<<endl<<endl<<endl<<endl;
return;
}
|