Although arrays greatly improved our ability to store data, there is
one major drawback to their use ... each element (each box) in an array must be of the
same data type. It is often desirable to group data of different types and work with that grouped data as one entity.
We now have the power to accomplish this grouping with a new data type called a
structure.
A structure is a collection of variable types grouped together.
You can refer to a structure as a single variable, and to its parts as
members of that variable by using the dot (.) operator.
The power of structures lies in the fact that once defined, the structure name becomes a
user-defined data type and may be used the same way as other built-in data
types, such as int, double,
char, and apstring.
Defining a structure
When dealing with the students in a college, many
variables of different types are needed. It may be necessary to keep track
of a name, an address (street, city, state, zip code), an age, an ID number, and a
grade point average, for example.
struct STUDENT_TYPE
{
apstring name, street, city, state, zipcode;
int age;
double IDnum;
double grade;
} ;
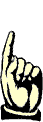 |
Note semicolon!!! |
The name of the
struct
is in capital letters followed by the word TYPE. While this naming
convention is NOT required, it may help you to remember that you have
created a new data type. |
STUDENT_TYPE is called the
structure tag,
and is
your brand new data type, like int,
double, char or apstring.
name, street, city, state, zipcode, age, IDnum,
and grade are structure members.
Declaring Variables of Type
struct
The most efficient method of dealing with structure variables is to define the structure
globally. This tells "the whole
world", namely main and any functions in the
program, that a new data type exists. To declare a structure globally,
place it BEFORE int main(void).
The structure variables can then be defined
locally in main,
for example.
. . .
struct STUDENT_TYPE
{
apstring name, street, city, state, zipcode;
int age;
double IDnum;
double grade;
} ;
int main(void)
{
// declare two variables of the new type
STUDENT_TYPE student1, student2;
// array of structures
apvector <STUDENT_TYPE> roster(10);
. . .
Alternate method of declaring variables of
type struct:
struct STUDENT_TYPE
{
apstring name, street, city, state, zipcode;
int age;
double IDnum;
double grade;
} student1, student2;
The variable names appear before the semicolon
ending the structure declaration. This method is useful
only when you know how many variables of this type your program
will be using. |
|