/*Once a structure has been defined, you can declare an apvector variable of that new data type.
This will create an array of structures.
In other words, instead of one cell of the array containing one piece of information,
one cell of the array will now contain all of the defined structure members.
*/
# include <iostream.h>
#include <apvector.h>
#include "apstring.cpp"
struct CD_TYPE //structure definition
{
apstring title, artist;
int year;
double cost;
} ;
int main(void)
{
apvector <CD_TYPE> collection(2); // declare an array of new data type
collection[0].title ="That's All Folks!"; //
drudge filling the structure for. . .
collection[0].artist = "Porky Pig"; // . . . the first cell of the array
collection[0].year = 2000;
collection[0].cost = 12.95;
collection[1].title ="I Thought I Saw A Putty Cat!"; //
drudge filling the structure for. . .
collection[1].artist = Tweety Bird;
// . . . the second cell of the array
collection[1].year = 2001;
collection[1].cost = 14.50;
// Prints the data
cout << "\n\n\nHere is the array of structures:\n\n";
for (int i = 0; i < collection.length(); i ++)
//notice that .length( ) still works
{
cout << "CD
Collection #" << i+1<<":\n";
cout << "Title: " <<
collection[i].title << endl;
cout << "Artist: " <<
collection[i].artist << endl;
cout << "Year: " <<
collection[i].year << endl;
cout<< "Cost:" <<
collection[i].cost <<"\n\n\n";
}
return 0;
}
A more realistic program would gather data by using a for loop or data file.
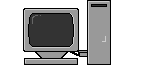
|